C Language
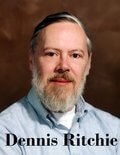
History of C Language
History of C language is interesting to know. Here we are going to discuss a brief history of the c language.
C programming language was developed in 1972 by Dennis Ritchie at bell laboratories of AT&T (American Telephone & Telegraph), located in the U.S.A.
Dennis Ritchie is known as the founder of the c language.
It was developed to overcome the problems of previous languages such as B, BCPL, etc.
Initially, C language was developed to be used in UNIX operating system. It inherits many features of previous languages such as B and BCPL.
Let's see the programming languages that were developed before C language.
Language | Year | Developed By |
---|---|---|
Algol | 1960 | International Group |
BCPL | 1967 | Martin Richard |
B | 1970 | Ken Thompson |
Traditional C | 1972 | Dennis Ritchie |
K & R C | 1978 | Kernighan & Dennis Ritchie |
ANSI C | 1989 | ANSI Committee |
ANSI/ISO C | 1990 | ISO Committee |
C99 | 1999 | Standardization Committee |
Features of C Language
C is the widely used language. It provides many featuresthat are given below.
- Simple
- Machine Independent or Portable
- Mid-level programming language
- structured programming language
- Rich Library
- Memory Management
- Fast Speed
- Pointers
- Recursion
- Extensible
1) Simple
C is a simple language in the sense that it provides a structured approach (to break the problem into parts), the rich set of library functions, data types, etc.
2) Machine Independent or Portable
Unlike assembly language, c programs can be executed on different machines with some machine specific changes. Therefore, C is a machine independent language.
3) Mid-level programming language
Although, C is intended to do low-level programming. It is used to develop system applications such as kernel, driver, etc. It also supports the features of a high-level language. That is why it is known as mid-level language.
4) Structured programming language
C is a structured programming language in the sense that we can break the program into parts using functions. So, it is easy to understand and modify. Functions also provide code reusability.
5) Rich Library
C provides a lot of inbuilt functions that make the development fast.
6) Memory Management
It supports the feature of dynamic memory allocation. In C language, we can free the allocated memory at any time by calling the free() function.
7) Speed
The compilation and execution time of C language is fast since there are lesser inbuilt functions and hence the lesser overhead.
8) Pointer
C provides the feature of pointers. We can directly interact with the memory by using the pointers. We can use pointers for memory, structures, functions, array, etc.
9) Recursion
In C, we can call the function within the function. It provides code reusability for every function. Recursion enables us to use the approach of backtracking.
10) Extensible
C language is extensible because it can easily adopt new features.
How to install C
There are many compilers available for c and c++. You need to download any one. Here, we are going to use Turbo C++. It will work for both C and C++. To install the Turbo C software, you need to follow following steps.
- Download Turbo C++
- Create turboc directory inside c drive and extract the tc3.zip inside c:\turboc
- Double click on install.exe file
- Click on the tc application file located inside c:\TC\BIN to write the c program
1) Download Turbo C++ software
You can download turbo c++ from many sites. download Turbo c++
2) Create turboc directory in c drive and extract the tc3.zip
Now, you need to create a new directory turboc inside the c: drive. Now extract the tc3.zip file in c:\truboc directory.
3) Double click on the install.exe file and follow steps
Now, click on the install icon located inside the c:\turboc
It will ask you to install c or not, press enter to install.
First C Program
Before starting the abcd of C language, you need to learn how to write, compile and run the first c program.
To write the first c program, open the C console and write the following code:
#include <stdio.h> includes the standard input output library functions. The printf() function is defined in stdio.h .
int main() The main() function is the entry point of every program in c language.
printf() The printf() function is used to print data on the console.
return 0 The return 0 statement, returns execution status to the OS. The 0 value is used for successful execution and 1 for unsuccessful execution.
How to compile and run the c program
There are 2 ways to compile and run the c program, by menu and by shortcut.
By menu
Now click on the compile menu then compile sub menu to compile the c program.
Then click on the run menu then run sub menu to run the c program.
By shortcut
Or, press ctrl+f9 keys compile and run the program directly.
You will see the following output on user screen.
Flow of C Program
The C program follows many steps in execution. To understand the flow of C program well, let us see a simple program first.
File: simple.c
Execution Flow
Let's try to understand the flow of above program by the figure given below.
1) C program (source code) is sent to preprocessor first. The preprocessor is responsible to convert preprocessor directives into their respective values. The preprocessor generates an expanded source code.
2) Expanded source code is sent to compiler which compiles the code and converts it into assembly code.
3) The assembly code is sent to assembler which assembles the code and converts it into object code. Now a simple.obj file is generated.
4) The object code is sent to linker which links it to the library such as header files. Then it is converted into executable code. A simple.exe file is generated.
5) The executable code is sent to loader which loads it into memory and then it is executed. After execution, output is sent to console.
The syntax of printf() function is given below:
The format string can be %d (integer), %c (character), %s (string), %f (float) etc.
printf() and scanf() in C
The printf() and scanf() functions are used for input and output in C language. Both functions are inbuilt library functions, defined in stdio.h (header file).printf() function
The printf() function is used for output. It prints the given statement to the console.The syntax of printf() function is given below:
scanf() function
The scanf() function is used for input. It reads the input data from the console.Program to print cube of given number
Let's see a simple example of c language that gets input from the user and prints the cube of the given number.Output
enter a number:5
cube of number is:125
The printf("cube of number is:%d ",number*number*number) statement prints the cube of number on the console.
Program to print sum of 2 numbers
Let's see a simple example of input and output in C language that prints addition of 2 numbers.Output
enter first number:15
enter second number:5
sum of 2 numbers:20
Variables in C
A variable is a name of the memory location. It is used to store data. Its value can be changed, and it can be reused many times.
It is a way to represent memory location through symbol so that it can be easily identified.
Let's see the syntax to declare a variable:
The example of declaring the variable is given below:
Here, a, b, c are variables. The int, float, char are the data types.
We can also provide values while declaring the variables as given below:
Rules for defining variables
- A variable can have alphabets, digits, and underscore.
- A variable name can start with the alphabet, and underscore only. It can't start with a digit.
- No whitespace is allowed within the variable name.
- A variable name must not be any reserved word or keyword, e.g. int, float, etc.
Valid variable names:
Invalid variable names:
Types of Variables in C
There are many types of variables in c:
- local variable
- global variable
- static variable
- automatic variable
- external variable
Local Variable
A variable that is declared inside the function or block is called a local variable.
It must be declared at the start of the block.
You must have to initialize the local variable before it is used.
Global Variable
A variable that is declared outside the function or block is called a global variable. Any function can change the value of the global variable. It is available to all the functions.
It must be declared at the start of the block.
Static Variable
A variable that is declared with the static keyword is called static variable.
It retains its value between multiple function calls.
If you call this function many times, the local variable will print the same value for each function call, e.g, 11,11,11 and so on. But the static variable will print the incremented value in each function call, e.g. 11, 12, 13 and so on.
Automatic Variable
All variables in C that are declared inside the block, are automatic variables by default. We can explicitly declare an automatic variable using auto keyword.
External Variable
We can share a variable in multiple C source files by using an external variable. To declare an external variable, you need to use extern keyword.
myfile.hData Types in C
A data type specifies the type of data that a variable can store such as integer, floating, character, etc.
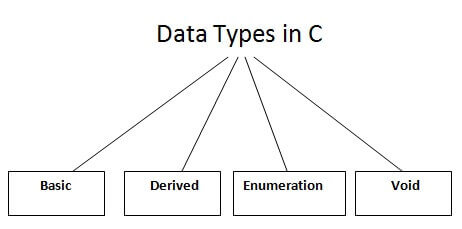
There are the following data types in C language.
Types | Data Types |
---|---|
Basic Data Type | int, char, float, double |
Derived Data Type | array, pointer, structure, union |
Enumeration Data Type | enum |
Void Data Type | void |
Data Types in CA data type specifies the type of data that a variable can store such as integer, floating, character, etc.![]()
The basic data types are integer-based and floating-point based. C language supports both signed and unsigned literals. |
The memory size of the basic data types may change according to 32 or 64-bit operating system.
Let's see the basic data types. Its size is given according to 32-bit architecture.
Data Types | Memory Size | Range |
---|---|---|
char | 1 byte | −128 to 127 |
signed char | 1 byte | −128 to 127 |
unsigned char | 1 byte | 0 to 255 |
short | 2 byte | −32,768 to 32,767 |
signed short | 2 byte | −32,768 to 32,767 |
unsigned short | 2 byte | 0 to 65,535 |
int | 2 byte | −32,768 to 32,767 |
signed int | 2 byte | −32,768 to 32,767 |
unsigned int | 2 byte | 0 to 65,535 |
short int | 2 byte | −32,768 to 32,767 |
signed short int | 2 byte | −32,768 to 32,767 |
unsigned short int | 2 byte | 0 to 65,535 |
long int | 4 byte | -2,147,483,648 to 2,147,483,647 |
signed long int | 4 byte | -2,147,483,648 to 2,147,483,647 |
unsigned long int | 4 byte | 0 to 4,294,967,295 |
float | 4 byte | |
double | 8 byte | |
long double | 10 byte |
Keywords in C
A keyword is a reserved word. You cannot use it as a variable name, constant name, etc. There are only 32 reserved words (keywords) in the C language.
A list of 32 keywords in the c language is given below:
auto | break | case | char | const | continue | default | do |
double | else | enum | extern | float | for | goto | if |
int | long | register | return | short | signed | sizeof | static |
struct | switch | typedef | union | unsigned | void | volatile | while |
We will learn about all the C language keywords later.
Keywords in C
A keyword is a reserved word. You cannot use it as a variable name, constant name, etc. There are only 32 reserved words (keywords) in the C language.
A list of 32 keywords in the c language is given below:
auto | break | case | char | const | continue | default | do |
double | else | enum | extern | float | for | goto | if |
int | long | register | return | short | signed | sizeof | static |
struct | switch | typedef | union | unsigned | void | volatile | while |
We will learn about all the C language keywords later.
C Operators
An operator is simply a symbol that is used to perform operations. There can be many types of operations like arithmetic, logical, bitwise, etc.
There are following types of operators to perform different types of operations in C language.
- Arithmetic Operators
- Relational Operators
- Shift Operators
- Logical Operators
- Bitwise Operators
- Ternary or Conditional Operators
- Assignment Operator
- Misc Operator
Precedence of Operators in C
The precedence of operator species that which operator will be evaluated first and next. The associativity specifies the operator direction to be evaluated; it may be left to right or right to left.- Let's understand the precedence by the example given below:
The precedence and associativity of C operators is given below:Category Operator Associativity Postfix () [] -> . ++ - - Left to right Unary + - ! ~ ++ - - (type)* & sizeof Right to left Multiplicative * / % Left to right Additive + - Left to right Shift << >> Left to right Relational < <= > >= Left to right Equality == != Left to right Bitwise AND & Left to right Bitwise XOR ^ Left to right Bitwise OR | Left to right Logical AND && Left to right Logical OR || Left to right Conditional ?: Right to left Assignment = += -= *= /= %=>>= <<= &= ^= |= Right to left Comma , Left to right
Comments in C
Comments in C language are used to provide information about lines of code. It is widely used for documenting code. There are 2 types of comments in the C language.
- Single Line Comments
- Multi-Line Comments
Single Line Comments
Single line comments are represented by double slash \\. Let's see an example of a single line comment in C.
Output:
Hello C
Even you can place the comment after the statement. For example:
Mult Line Comments
Multi-Line comments are represented by slash asterisk \* ... *\. It can occupy many lines of code, but it can't be nested. Syntax:
Let's see an example of a multi-Line comment in C.
Output:
Hello C
Escape Sequence in C
An escape sequence in C language is a sequence of characters that doesn't represent itself when used inside string literal or character.
It is composed of two or more characters starting with backslash \. For example: \n represents new line.
List of Escape Sequences in C
Escape Sequence | Meaning |
---|---|
\a | Alarm or Beep |
\b | Backspace |
\f | Form Feed |
\n | New Line |
\r | Carriage Return |
\t | Tab (Horizontal) |
\v | Vertical Tab |
\\ | Backslash |
\' | Single Quote |
\" | Double Quote |
\? | Question Mark |
\nnn | octal number |
\xhh | hexadecimal number |
\0 | Null |
Escape Sequence Example
Output:You
are
learning
'c' language
"Do you know C language"
Constants in C
A constant is a value or variable that can't be changed in the program, for example: 10, 20, 'a', 3.4, "c programming" etc.There are different types of constants in C programming.List of Constants in C
Constant | Example |
---|---|
Decimal Constant | 10, 20, 450 etc. |
Real or Floating-point Constant | 10.3, 20.2, 450.6 etc. |
Octal Constant | 021, 033, 046 etc. |
Hexadecimal Constant | 0x2a, 0x7b, 0xaa etc. |
Character Constant | 'a', 'b', 'x' etc. |
String Constant | "c", "c program", "c in javatpoint" etc. |
Constants in C
A constant is a value or variable that can't be changed in the program, for example: 10, 20, 'a', 3.4, "c programming" etc.
There are different types of constants in C programming.
List of Constants in C
There are two ways to define constant in C programming.
|
- const keyword
- #define preprocessor
1) C const keyword
The const keyword is used to define constant in C programming.
Now, the value of PI variable can't be changed.
Output:
The value of PI is: 3.140000
If you try to change the the value of PI, it will render compile time error.
Output:
Compile Time Error: Cannot modify a const object
2) C #define preprocessor
The #define preprocessor is also used to define constant. We will learn about #define preprocessor directive later.
Visit here for: #define preprocessor directive.
C if else Statement
The if-else statement in C is used to perform the operations based on some specific condition. The operations specified in if block are executed if and only if the given condition is true.
There are the following variants of if statement in C language.
- If statement
- If-else statement
- If else-if ladder
- Nested if
If Statement
The if statement is used to check some given condition and perform some operations depending upon the correctness of that condition. It is mostly used in the scenario where we need to perform the different operations for the different conditions. The syntax of the if statement is given below.
Flowchart of if statement in C

Let's see a simple example of C language if statement.
Output
Enter a number:4
4 is even number
enter a number:5
Program to find the largest number of the three.
Output
Enter three numbers?
12 23 34
34 is largest
If-else Statement
The if-else statement is used to perform two operations for a single condition. The if-else statement is an extension to the if statement using which, we can perform two different operations, i.e., one is for the correctness of that condition, and the other is for the incorrectness of the condition. Here, we must notice that if and else block cannot be executed simiulteneously. Using if-else statement is always preferable since it always invokes an otherwise case with every if condition. The syntax of the if-else statement is given below.
Flowchart of the if-else statement in C
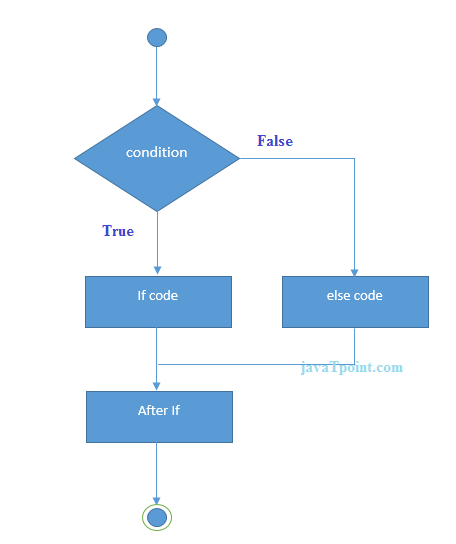
Let's see the simple example to check whether a number is even or odd using if-else statement in C language.
Output
enter a number:4
4 is even number
enter a number:5
5 is odd number
Program to check whether a person is eligible to vote or not.
Output
Enter your age?18
You are eligible to vote...
Enter your age?13
Sorry ... you can't vote
If else-if ladder Statement
The if-else-if ladder statement is an extension to the if-else statement. It is used in the scenario where there are multiple cases to be performed for different conditions. In if-else-if ladder statement, if a condition is true then the statements defined in the if block will be executed, otherwise if some other condition is true then the statements defined in the else-if block will be executed, at the last if none of the condition is true then the statements defined in the else block will be executed. There are multiple else-if blocks possible. It is similar to the switch case statement where the default is executed instead of else block if none of the cases is matched.
Flowchart of else-if ladder statement in C
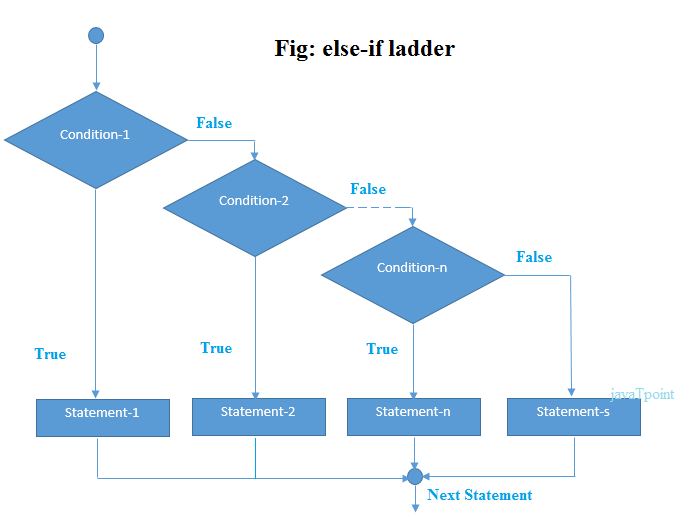
The example of an if-else-if statement in C language is given below.
Output
enter a number:4
number is not equal to 10, 50 or 100
enter a number:50
number is equal to 50
Program to calculate the grade of the student according to the specified marks.
Output
Enter your marks?10
Sorry you are fail ...
Enter your marks?40
You scored grade C ...
Enter your marks?90
Congrats ! you scored grade A ...
C Switch Statement
The switch statement in C is an alternate to if-else-if ladder statement which allows us to execute multiple operations for the different possibles values of a single variable called switch variable. Here, We can define various statements in the multiple cases for the different values of a single variable.
The syntax of switch statement in c language is given below:
C Loops
The looping can be defined as repeating the same process multiple times until a specific condition satisfies. There are three types of loops used in the C language. In this part of the tutorial, we are going to learn all the aspects of C loops.
Why use loops in C language?
The looping simplifies the complex problems into the easy ones. It enables us to alter the flow of the program so that instead of writing the same code again and again, we can repeat the same code for a finite number of times. For example, if we need to print the first 10 natural numbers then, instead of using the printf statement 10 times, we can print inside a loop which runs up to 10 iterations.
Advantage of loops in C
1) It provides code reusability.
2) Using loops, we do not need to write the same code again and again.
2) Using loops, we can traverse over the elements of data structures (array or linked lists).
Types of C Loops
There are three types of loops in C language that is given below:
- do while
- while
- for
do-while loop in C
The do-while loop continues until a given condition satisfies. It is also called post tested loop. It is used when it is necessary to execute the loop at least once (mostly menu driven programs).
The syntax of do-while loop in c language is given below:
while loop in C
The while loop in c is to be used in the scenario where we don't know the number of iterations in advance. The block of statements is executed in the while loop until the condition specified in the while loop is satisfied. It is also called a pre-tested loop.
The syntax of while loop in c language is given below:
for loop in C
The for loop is used in the case where we need to execute some part of the code until the given condition is satisfied. The for loop is also called as a per-tested loop. It is better to use for loop if the number of iteration is known in advance.
The syntax of for loop in c language is given below:
do while loop in C
The do while loop is a post tested loop. Using the do-while loop, we can repeat the execution of several parts of the statements. The do-while loop is mainly used in the case where we need to execute the loop at least once. The do-while loop is mostly used in menu-driven programs where the termination condition depends upon the end user.
do while loop syntax
The syntax of the C language do-while loop is given below:
Example 1
Output
1. Print Hello
2. Print Javatpoint
3. Exit
1
Hello
do you want to enter more?
y
1. Print Hello
2. Print Javatpoint
3. Exit
2
Javatpoint
do you want to enter more?
n
Flowchart of do while loop
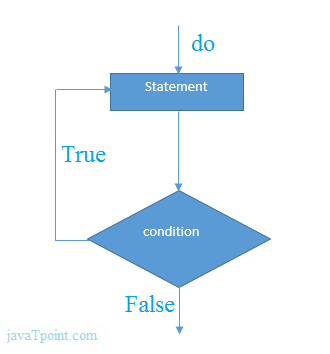
do while loop in CThe do while loop is a post tested loop. Using the do-while loop, we can repeat the execution of several parts of the statements. The do-while loop is mainly used in the case where we need to execute the loop at least once. The do-while loop is mostly used in menu-driven programs where the termination condition depends upon the end user.do while loop syntaxThe syntax of the C language do-while loop is given below:Example 1Output1. Print Hello
2. Print Javatpoint
3. Exit
1
Hello
do you want to enter more?
y
1. Print Hello
2. Print Javatpoint
3. Exit
2
Javatpoint
do you want to enter more?
n
Flowchart of do while loop![]() do while exampleThere is given the simple program of c language do while loop where we are printing the table of 1.Output1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using do while loopOutputEnter a number: 5
5
10
15
20
25
30
35
40
45
50
Enter a number: 10
10
20
30
40
50
60
70
80
90
100
Infinitive do while loopThe do-while loop will run infinite times if we pass any non-zero value as the conditional expression. |
while loop in C
While loop is also known as a pre-tested loop. In general, a while loop allows a part of the code to be executed multiple times depending upon a given boolean condition. It can be viewed as a repeating if statement. The while loop is mostly used in the case where the number of iterations is not known in advance.
Syntax of while loop in C language
The syntax of while loop in c language is given below:
Flowchart of while loop in C
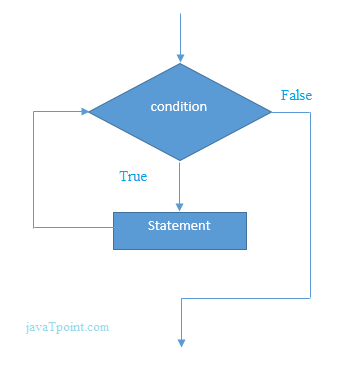
Example of the while loop in C language
Let's see the simple program of while loop that prints table of 1.
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using while loop in C
Output
Enter a number: 50
50
100
150
200
250
300
350
400
450
500
Enter a number: 100
100
200
300
400
500
600
700
800
900
1000
Properties of while loop
- A conditional expression is used to check the condition. The statements defined inside the while loop will repeatedly execute until the given condition fails.
- The condition will be true if it returns 0. The condition will be false if it returns any non-zero number.
- In while loop, the condition expression is compulsory.
- Running a while loop without a body is possible.
- We can have more than one conditional expression in while loop.
- If the loop body contains only one statement, then the braces are optional.
Example 1
Output
3 5 7 9 11
Example 2
Output
compile time error: while loop can't be empty
Example 3
Output
infinite loop
Infinitive while loop in C
If the expression passed in while loop results in any non-zero value then the loop will run the infinite number of times.
for loop in C
The for loop in C language is used to iterate the statements or a part of the program several times. It is frequently used to traverse the data structures like the array and linked list.
Syntax of for loop in C
The syntax of for loop in c language is given below:
Flowchart of for loop in C

C for loop Examples
Let's see the simple program of for loop that prints table of 1.
Output
1
2
3
4
5
6
7
8
9
10
C Program: Print table for the given number using C for loop
Output
Enter a number: 2
2
4
6
8
10
12
14
16
18
20
Enter a number: 1000
1000
2000
3000
4000
5000
6000
7000
8000
9000
10000
Properties of Expression 1
- The expression represents the initialization of the loop variable.
- We can initialize more than one variable in Expression 1.
- Expression 1 is optional.
- In C, we can not declare the variables in Expression 1. However, It can be an exception in some compilers.
Example 1
Output
35 36
Example 2
Output
1 2 3 4
Properties of Expression 2
- Expression 2 is a conditional expression. It checks for a specific condition to be satisfied. If it is not, the loop is terminated.
- Expression 2 can have more than one condition. However, the loop will iterate until the last condition becomes false. Other conditions will be treated as statements.
- Expression 2 is optional.
- Expression 2 can perform the task of expression 1 and expression 3. That is, we can initialize the variable as well as update the loop variable in expression 2 itself.
- We can pass zero or non-zero value in expression 2. However, in C, any non-zero value is true, and zero is false by default.
Example 1
output
0 1 2 3 4
Example 2
Output
0 0 0
1 2 3
2 4 6
3 6 9
4 8 12
Example 3
Output
infinite loop
Properties of Expression 3
- Expression 3 is used to update the loop variable.
- We can update more than one variable at the same time.
- Expression 3 is optional.
Example 1
Output
0 2
1 4
2 6
3 8
4 10
Loop body
The braces {} are used to define the scope of the loop. However, if the loop contains only one statement, then we don't need to use braces. A loop without a body is possible. The braces work as a block separator, i.e., the value variable declared inside for loop is valid only for that block and not outside. Consider the following example.
Output
20 20 20 20 20 20 20 20 20 20
Infinitive for loop in C
To make a for loop infinite, we need not give any expression in the syntax. Instead of that, we need to provide two semicolons to validate the syntax of the for loop. This will work as an infinite for loop.
If you run this program, you will see above statement infinite times.
C break statement
The break is a keyword in C which is used to bring the program control out of the loop. The break statement is used inside loops or switch statement. The break statement breaks the loop one by one, i.e., in the case of nested loops, it breaks the inner loop first and then proceeds to outer loops. The break statement in C can be used in the following two scenarios:
- With switch case
- With loop
Syntax:
Flowchart of break in c
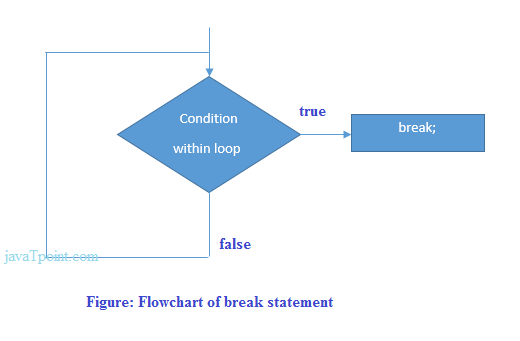
Example
Output
0 1 2 3 4 5 came outside of loop i = 5
Example of C break statement with switch case
Click here to see the example of C break with the switch statement.
C break statement with the nested loop
In such case, it breaks only the inner loop, but not outer loop.
Output
1 1
1 2
1 3
2 1
2 2
3 1
3 2
3 3
As you can see the output on the console, 2 3 is not printed because there is a break statement after printing i==2 and j==2. But 3 1, 3 2 and 3 3 are printed because the break statement is used to break the inner loop only.
break statement with while loop
Consider the following example to use break statement inside while loop.
Output
0 1 2 3 4 5 6 7 8 9 came out of while loop
break statement with do-while loop
Consider the following example to use the break statement with a do-while loop.
Output
2 X 1 = 2
2 X 2 = 4
2 X 3 = 6
2 X 4 = 8
2 X 5 = 10
2 X 6 = 12
2 X 7 = 14
2 X 8 = 16
2 X 9 = 18
2 X 10 = 20
do you want to continue with the table of 3 , enter any non-zero value to continue.1
3 X 1 = 3
3 X 2 = 6
3 X 3 = 9
3 X 4 = 12
3 X 5 = 15
3 X 6 = 18
3 X 7 = 21
3 X 8 = 24
3 X 9 = 27
3 X 10 = 30
do you want to continue with the table of 4 , enter any non-zero value to continue.0
C continue statementThe continue statement in C language is used to bring the program control to the beginning of the loop. The continue statement skips some lines of code inside the loop and continues with the next iteration. It is mainly used for a condition so that we can skip some code for a particular condition.Syntax:Continue statement example 1Output infinite loop
Continue statement example 2Output1
2
3
4
6
7
8
9
10
C continue statement with inner loopIn such case, C continue statement continues only inner loop, but not outer loop.Output1 1
1 2
1 3
2 1
2 3
3 1
3 2
3 3
|
Type Casting in C
Typecasting allows us to convert one data type into other. In C language, we use cast operator for typecasting which is denoted by (type).
Syntax:
Note: It is always recommended to convert the lower value to higher for avoiding data loss.
Without Type Casting:
With Type Casting:
Type Casting example
Let's see a simple example to cast int value into the float.
Output:
f : 2.250000
C Functions
In c, we can divide a large program into the basic building blocks known as function. The function contains the set of programming statements enclosed by {}. A function can be called multiple times to provide reusability and modularity to the C program. In other words, we can say that the collection of functions creates a program. The function is also known as procedure or subroutine in other programming languages.
Advantage of functions in C
There are the following advantages of C functions.
- By using functions, we can avoid rewriting same logic/code again and again in a program.
- We can call C functions any number of times in a program and from any place in a program.
- We can track a large C program easily when it is divided into multiple functions.
- Reusability is the main achievement of C functions.
- However, Function calling is always a overhead in a C program.
Function Aspects
There are three aspects of a C function.- Function declaration A function must be declared globally in a c program to tell the compiler about the function name, function parameters, and return type.
- Function call Function can be called from anywhere in the program. The parameter list must not differ in function calling and function declaration. We must pass the same number of functions as it is declared in the function declaration.
- Function definition It contains the actual statements which are to be executed. It is the most important aspect to which the control comes when the function is called. Here, we must notice that only one value can be returned from the function.
SN C function aspects Syntax 1 Function declaration return_type function_name (argument list); 2 Function call function_name (argument_list) 3 Function definition return_type function_name (argument list) {function body;} Types of Functions
There are two types of functions in C programming:- Library Functions: are the functions which are declared in the C header files such as scanf(), printf(), gets(), puts(), ceil(), floor() etc.
- User-defined functions: are the functions which are created by the C programmer, so that he/she can use it many times. It reduces the complexity of a big program and optimizes the code.
Return Value
A C function may or may not return a value from the function. If you don't have to return any value from the function, use void for the return type.
Let's see a simple example of C function that doesn't return any value from the function.
Example without return value:
Let's see a simple example of C function that returns int value from the function.
Example with return value:
Different aspects of function calling
A function may or may not accept any argument. It may or may not return any value. Based on these facts, There are four different aspects of function calls.- function without arguments and without return value
- function without arguments and with return value
- function with arguments and without return value
- function with arguments and with return value
Example for Function without argument and return value
Example 1
Hello Javatpoint
Example 2
Going to calculate the sum of two numbers: Enter two numbers 10 24 The sum is 34
Example for Function without argument and with return value
Example 1
Going to calculate the sum of two numbers: Enter two numbers 10 24 The sum is 34
Example 2: program to calculate the area of the square
Going to calculate the area of the square Enter the length of the side in meters: 10 The area of the square: 100.000000
Example for Function with argument and without return value
Example 1
Going to calculate the sum of two numbers: Enter two numbers 10 24 The sum is 34
Example 2: program to calculate the average of five numbers.
Going to calculate the average of five numbers: Enter five numbers:10 20 30 40 50 The average of given five numbers : 30.000000
Example for Function with argument and with return value
Example 1
Going to calculate the sum of two numbers: Enter two numbers:10 20 The sum is : 30
Example 2: Program to check whether a number is even or odd
Going to check whether a number is even or odd Enter the number: 100 The number is even
C Library Functions
Library functions are the inbuilt function in C that are grouped and placed at a common place called the library. Such functions are used to perform some specific operations. For example, printf is a library function used to print on the console. The library functions are created by the designers of compilers. All C standard library functions are defined inside the different header files saved with the extension .h. We need to include these header files in our program to make use of the library functions defined in such header files. For example, To use the library functions such as printf/scanf we need to include stdio.h in our program which is a header file that contains all the library functions regarding standard input/output.
The list of mostly used header files is given in the following table.SN Header file Description 1 stdio.h This is a standard input/output header file. It contains all the library functions regarding standard input/output. 2 conio.h This is a console input/output header file. 3 string.h It contains all string related library functions like gets(), puts(),etc. 4 stdlib.h This header file contains all the general library functions like malloc(), calloc(), exit(), etc. 5 math.h This header file contains all the math operations related functions like sqrt(), pow(), etc. 6 time.h This header file contains all the time-related functions. 7 ctype.h This header file contains all character handling functions. 8 stdarg.h Variable argument functions are defined in this header file. 9 signal.h All the signal handling functions are defined in this header file. 10 setjmp.h This file contains all the jump functions. 11 locale.h This file contains locale functions. 12 errno.h This file contains error handling functions. 13 assert.h This file contains diagnostics functions.
Call by value and Call by reference in C
There are two methods to pass the data into the function in C language, i.e., call by value and call by reference.
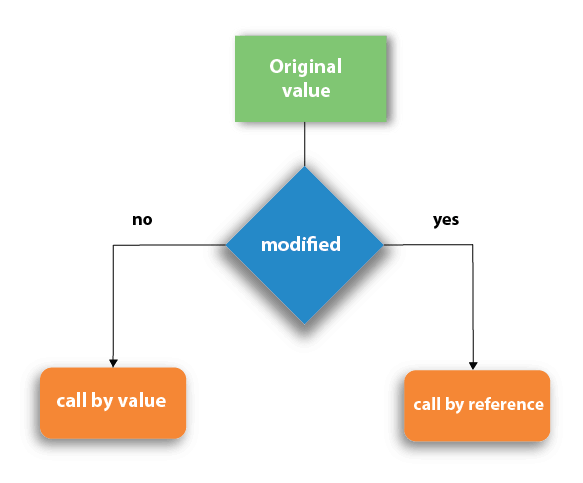
Let's understand call by value and call by reference in c language one by one.
Call by value in C
- In call by value method, the value of the actual parameters is copied into the formal parameters. In other words, we can say that the value of the variable is used in the function call in the call by value method.
- In call by value method, we can not modify the value of the actual parameter by the formal parameter.
- In call by value, different memory is allocated for actual and formal parameters since the value of the actual parameter is copied into the formal parameter.
- The actual parameter is the argument which is used in the function call whereas formal parameter is the argument which is used in the function definition.
Let's try to understand the concept of call by value in c language by the example given below:
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=100
Call by Value Example: Swapping the values of the two variables
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 10, b = 20
Call by reference in C
- In call by reference, the address of the variable is passed into the function call as the actual parameter.
- The value of the actual parameters can be modified by changing the formal parameters since the address of the actual parameters is passed.
- In call by reference, the memory allocation is similar for both formal parameters and actual parameters. All the operations in the function are performed on the value stored at the address of the actual parameters, and the modified value gets stored at the same address.
Consider the following example for the call by reference.
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=200
Call by reference Example: Swapping the values of the two variables
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 20, b = 10
Difference between call by value and call by reference in c
No. | Call by value | Call by reference |
---|---|---|
1 | A copy of the value is passed into the function | An address of value is passed into the function |
2 | Changes made inside the function is limited to the function only. The values of the actual parameters do not change by changing the formal parameters. | Changes made inside the function validate outside of the function also. The values of the actual parameters do change by changing the formal parameters. |
3 | Actual and formal arguments are created at the different memory location | Actual and formal arguments are created at the same memory location |
Recursion in C
Recursion is the process which comes into existence when a function calls a copy of itself to work on a smaller problem. Any function which calls itself is called recursive function, and such function calls are called recursive calls. Recursion involves several numbers of recursive calls. However, it is important to impose a termination condition of recursion. Recursion code is shorter than iterative code however it is difficult to understand.
Recursion cannot be applied to all the problem, but it is more useful for the tasks that can be defined in terms of similar subtasks. For Example, recursion may be applied to sorting, searching, and traversal problems.
Generally, iterative solutions are more efficient than recursion since function call is always overhead. Any problem that can be solved recursively, can also be solved iteratively. However, some problems are best suited to be solved by the recursion, for example, tower of Hanoi, Fibacci , factorial findinong, etc.In the following example, recursion is used to calculate the factorial of a number.
Output
Enter the number whose factorial you want to calculate?5 factorial = 120
We can understand the above program of the recursive method call by the figure given below: